How to Check if a File Exists in PHP
In PHP development, file handling is essential for creating dynamic and interactive applications. One important aspect of file handling is checking whether a file exists before attempting to access or modify it. Failing to do this can result in errors or unexpected behavior in your application.
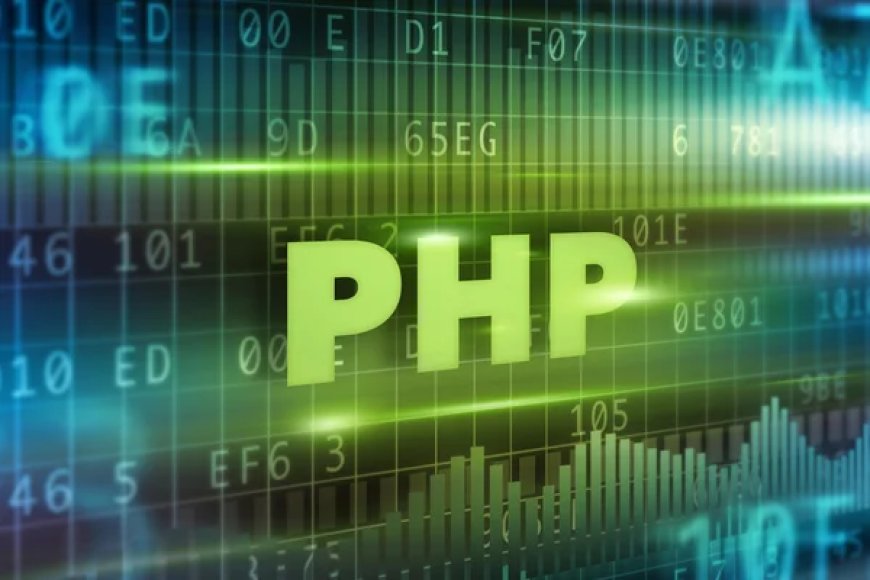
In PHP development, file handling is essential for creating dynamic and interactive applications. One important aspect of file handling is checking whether a file exists before attempting to access or modify it. Failing to do this can result in errors or unexpected behavior in your application.
Why Check if a File Exists?
Ensuring a file exists before interacting with it has several benefits:
- Error Prevention: Helps prevent file-not-found errors, which can disrupt the functionality of your application.
- User Experience: Provides a seamless experience by handling missing files gracefully.
- Security: Reduces the risk of security vulnerabilities associated with file operations.
Using file_exists()
Function in PHP
The most common way to check if a file exists in PHP is by using the file_exists()
function. This function returns true
if the specified file exists and false
otherwise.
Syntax:
Example:
if (file_exists("example.txt")) {
echo "File exists.";
} else {
echo "File does not exist.";
}
Checking for Files in Different Locations
To use file_exists()
effectively, understanding absolute and relative paths is crucial.
- Absolute Path: Refers to the full path from the root directory (e.g.,
/var/www/html/example.txt
). - Relative Path: Refers to the path relative to the current working directory (e.g.,
../example.txt
).
Example:
Using is_file()
for File Validation
While file_exists()
checks if a file or directory exists, is_file()
checks specifically for files.
Syntax:
is_file(string $filename): bool
Example:
if (is_file("data.json")) {
echo "This is a file.";
} else {
echo "Not a file.";
}
Error Handling When Checking for Files
PHP doesn’t throw exceptions when a file doesn’t exist, but handling errors gracefully is a good practice.
Example:
try {
if (file_exists("nonexistent.txt")) {
// Proceed with file handling
} else {
throw new Exception("File not found.");
}
} catch (Exception $e) {
echo $e->getMessage();
}
Checking Directory Existence with is_dir()
To verify if a directory exists, use the is_dir()
function, which works similarly to file_exists()
but is specific to directories.
Example:
Using fopen()
for Advanced File Handling
The fopen()
function can also be used to check for file existence indirectly, as it will return false
if a file cannot be opened.
Example:
$file = @fopen("data.txt", "r");
if ($file) {
echo "File opened successfully.";
fclose($file);
} else {
echo "File does not exist or cannot be opened.";
}
Best Practices for Checking Files in PHP
- Avoid redundant file checks by storing results in variables.
- Ensure file paths are sanitized to prevent path traversal vulnerabilities.
Using PHP Constants and Functions for Paths
PHP offers constants like __DIR__
and __FILE__
to help with file paths. These are particularly useful for relative paths.
Example:
$filePath = __DIR__ . "/logs/error.log";
if (file_exists($filePath)) {
echo "Log file exists.";
}
Alternative PHP Functions for File Handling
Apart from file_exists()
, PHP offers other functions like stat()
and glob()
that can be used for file and directory operations.
Performance Considerations
Avoid excessive file checks within loops as they may impact performance. When possible, consolidate file operations to reduce server load.
Common Use Cases for File Existence Check
Checking file existence is especially useful in applications that handle:
- Image uploads
- Document downloads
- Log file management
Code Snippets and Examples
Here’s a simple function to check for a file and create it if it doesn’t exist:
Conclusion
Checking if a file exists is essential for reliable file handling in PHP. By using functions like file_exists()
, is_file()
, and is_dir()
, you can create robust applications that handle file operations smoothly and securely.
What's Your Reaction?






